A Developer’s Guide to Python Unit Testing
"If it works, don’t touch it" is a common mantra for developers (especially juniors). While this approach works in static environments, software rarely stays unchanged.
Unit testing ensures everything works as it should. A survey of developers revealed that developers understand the importance of testing, but due to time constraints and resources, it doesn't always happen.
Automated testing is one way out of it - but before you do that, you need to understand what it is you’re automating and why.
Table of Contents
Unit testing is writing test cases that verify the behavior and correctness of specific code units, such as functions, methods, or classes.
Unit tests enhance code quality, reduce debugging time, and boost confidence in software reliability. By testing individual components separately, you can catch and fix bugs early in the development process before they propagate and become more challenging to identify and resolve.
Writing unit tests forces you to think about the design and behavior of your code. When you write a unit test, you must consider -
- the input and output of a specific function
- any edge cases
- and how you would like errors handled.
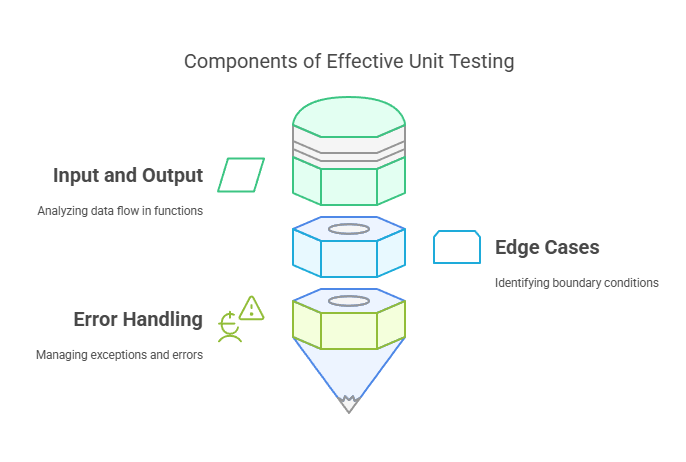
Components of Effective Unit Testing
Unit testing saves time and effort by streamlining debugging and improving troubleshooting efficiency:
- Simplifies debugging with pre-written test cases.
- Pinpoints issues in specific functions or methods.
- Reduces guesswork during troubleshooting.
- Eliminates the need to search the entire codebase.
- Improves overall development efficiency and code reliability.
Unit tests provide a safety net by ensuring code changes don’t introduce bugs or break features. Failed tests highlight issues, enabling confident refactoring and reducing side effects by catching unintended behavior. The process improves code reliability and makes future development more efficient. Adopting a 'born left' security approach can further enhance code quality by integrating security measures from the inception of the development process.
Developers often encounter challenges when writing unit tests that can hinder their progress. Here are some of the most common challenges developers face.
1. Writing Tests Is Time-Consuming
Writing unit tests is time-intensive, especially as the codebase grows.
Example:
- Functions: 10
- Test cases per function: 8
- Time per test case: 0.5 hours
Formula:
Total Time = Functions × Test Cases × Time per Case
Calculation:
10 × 8 × 0.5 = 40 hours

Why Devs Don’t Like Testing
2. Gaps in Test Coverage
Gaps in test coverage can lead to undetected bugs or undesired code behavior.
Tools like coverage.py help address test coverage gaps by identifying untested code during test execution. It provides visual reports on code coverage and integrates well with frameworks like unittest and pytest, simplifying its adoption in your workflow.
Coverage reports show which parts of your code were executed during tests and highlight untested areas, helping ensure comprehensive test coverage.
3. Maintaining Tests Can Be Challenging
Tests are still code and must be maintained just like any software. As requirements change, previously written tests can break, adding a maintenance layer.
Similarly, updating these tests is time-consuming. As developers, whenever we encounter a repetitive task, the natural solution is to automate it. Tools like EarlyAI integrate with Visual Studio Code and can generate and maintain validated unit tests for your Python projects.
Here are steps to help you get started with your Python unit tests.
Step 1: Choose the Right Testing Framework
The first step in writing Python unit tests is selecting a suitable framework. Popular options include unittest, pytest, and nose2, each with unique strengths and use cases.
Unittest
- Built-in Python framework, no dependencies needed.
- Approach: Class-based, structured.
- Key Features: Test cases, suites, runners for traditional, systematic testing.
- Best For: Conventional, structured projects.
Pytest
- Open-source, known for simplicity and readability.
- Approach: Function-based, concise syntax.
- Key Features: Plugin ecosystem (e.g., coverage, parallel execution), expressive syntax.
- Best For: Flexible, easy-to-use projects.
Nose2
- Successor to nose; compatible with unittest.
- Approach: Unittest-compatible, extensible.
- Key Features: Test discovery, support for complex needs.
- Best For: Projects needing unittest compatibility and extensibility
Step 2: Write Your First Unit Test
In this step, we'll walk through a simple example using the unittest framework. We'll create an essential function and write a corresponding unit test to verify its behavior.
Suppose you have a function called is_valid_email that checks whether a given string is a valid email address.
Here's how the is_valid_email function might look:
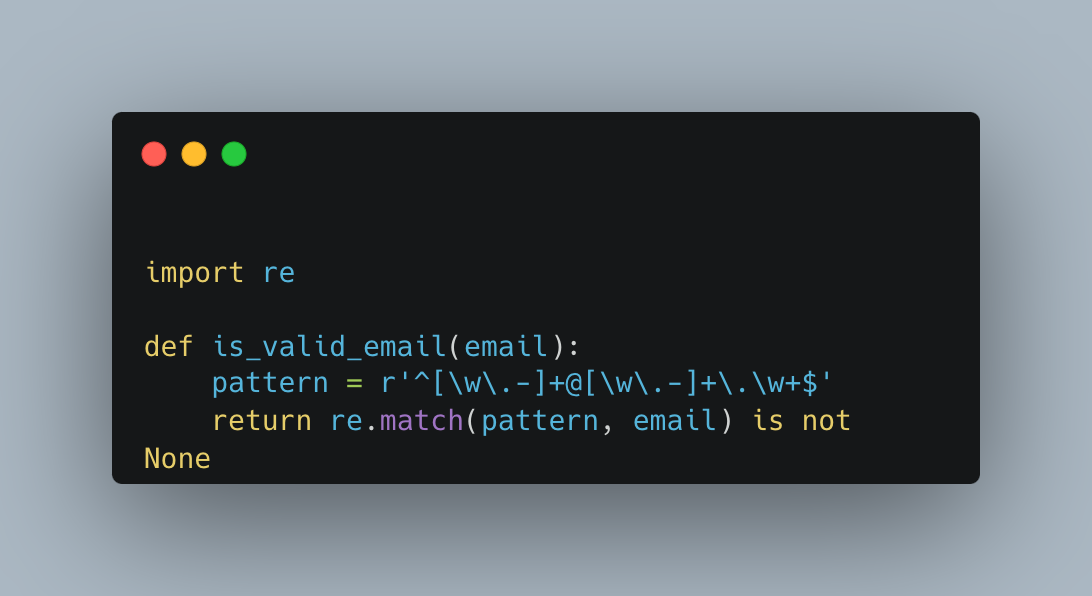
Unit test example
This function uses a regular expression to validate email format, returning True for valid emails and False otherwise. To test it, create a file named test_email_validation.py and add the following code:
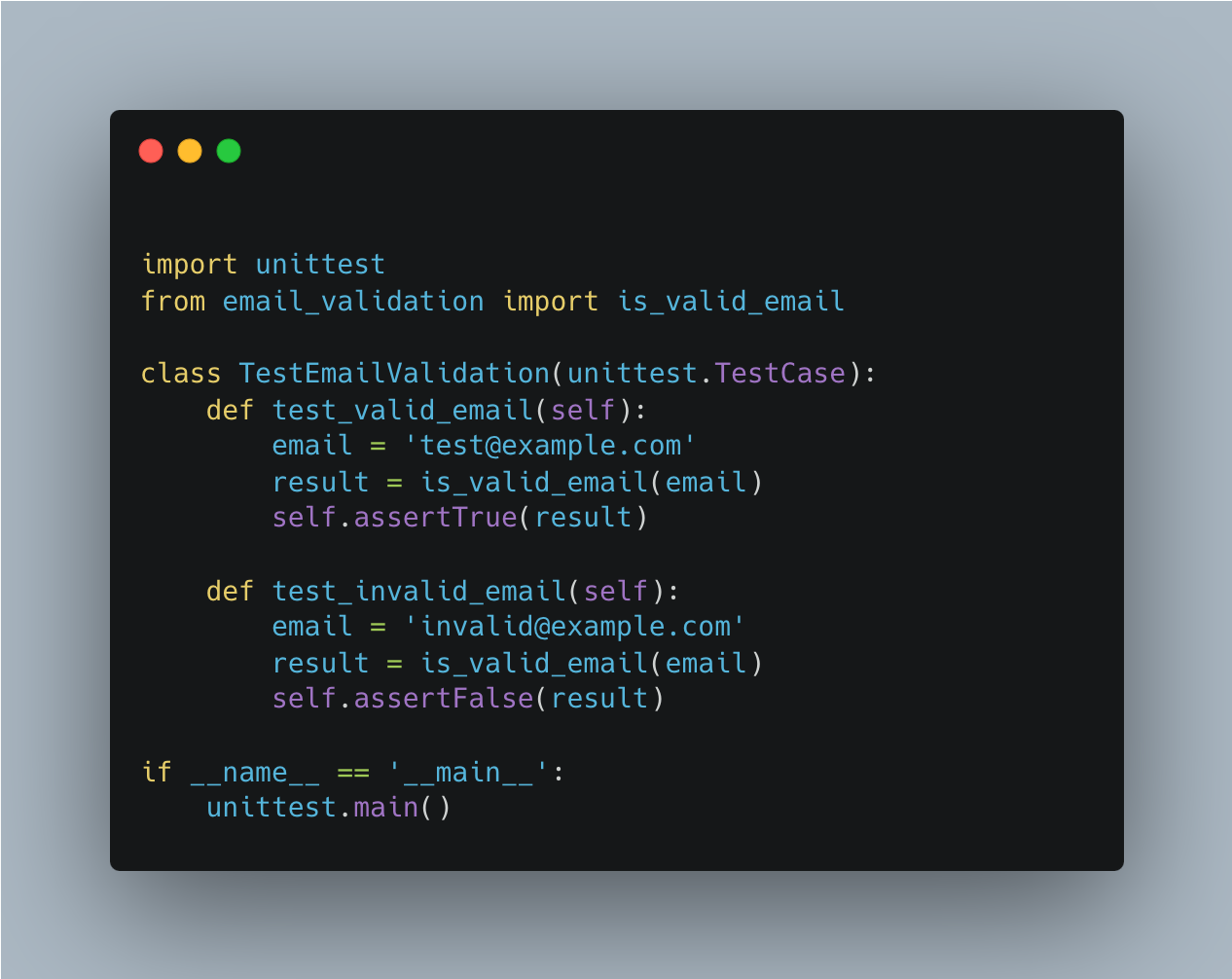
TestEmailValidation class
The test file includes a TestEmailValidation class inheriting from unittest with two methods:
- test_valid_email: Tests if is_valid_email correctly identifies a valid email using assertTrue.
- test_invalid_email: Tests if is_valid_email correctly flags an invalid email using assertFalse.
Step 3: Run and Debug Your Tests
To run the tests for our is_valid_email function, execute the following command:
python test_email_validation.py
Output is similar to:

Test output
The dots indicate that both tests passed successfully.
Next, let's introduce an error in our is_valid_email function to see how test failures are reported. Modify the function as follows:

Email validation python unit test
We added a case-insensitive condition to invalidate emails containing "test." Rerun the tests using the command:
python test_email_validation.py
Output is similar to:
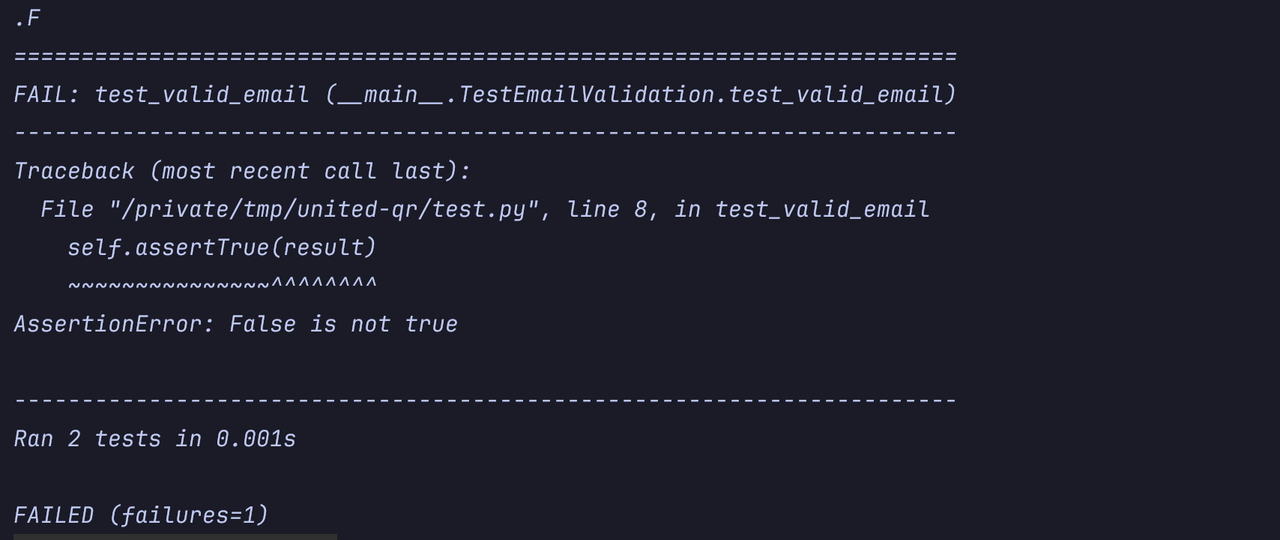
Email validation test results
The report indicates that the test_valid_email test failed because the is_valid_email function considered the email invalid, even though the test expected it to be valid.
To fix the failing test, you can modify the is_valid_email function to remove the additional condition or add a test case to account for this change.
Step 4: Organize Your Test Files
Keeping your test files organized and maintainable is essential. Proper organization helps you easily navigate and manage your test suite, making running tests and tracking down issues more efficient.
Organize your test files in a way that mirrors the structure of your project.
project/
├── src/
│ ├── module1.py
│ └── module2.py
└── tests/
├── test_module1.py
└── test_module2.py
Best practices exist to save you time. Here are the top 5 when it comes to Python unit testing.
1. Use descriptive file names
Name your test files in a manner that indicates what they are testing.
A standard convention is to prefix the test file names with test_ followed by the module's name or functionality being tested.
Eg:
Your module → email_validation.py
Your test → test_email_validation.py
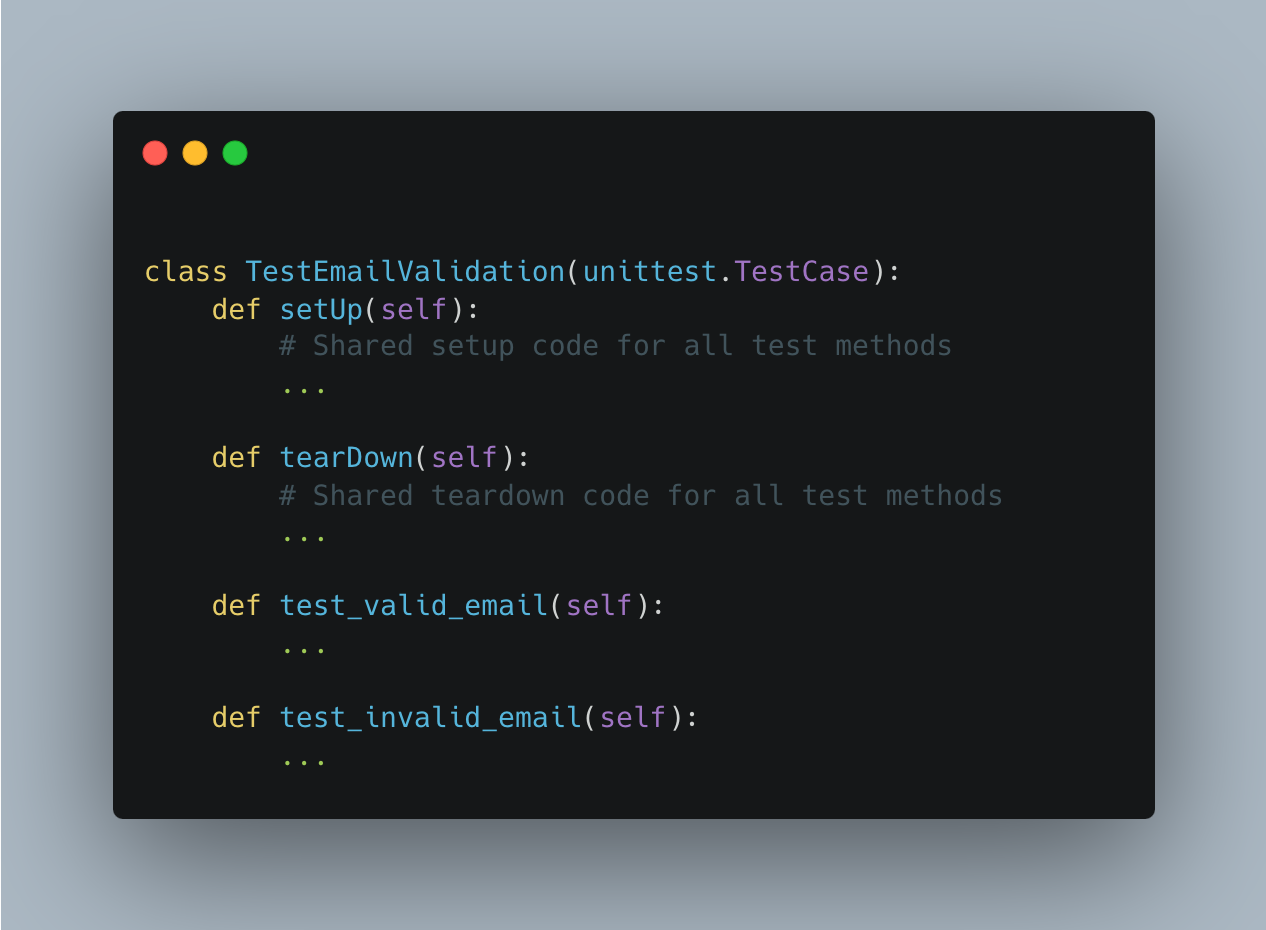
Python email validation test class
2. Use Mocking and Stubbing for Isolation
Use Mocking and Stubbing for Isolation, and apply role-based access control (RBAC) to protect test environments.
Mocking and stubbing are techniques for simulating the behavior of external dependencies during testing. They allow you to replace real objects or functions with fake or predefined ones, giving you control over their behavior and output.
3. Leverage parameterized tests for Efficiency
With features like pytest.mark.parametrize, you can run a single test case with multiple input combinations. The approach saves time, reduces redundant code, and ensures comprehensive test coverage across various scenarios.
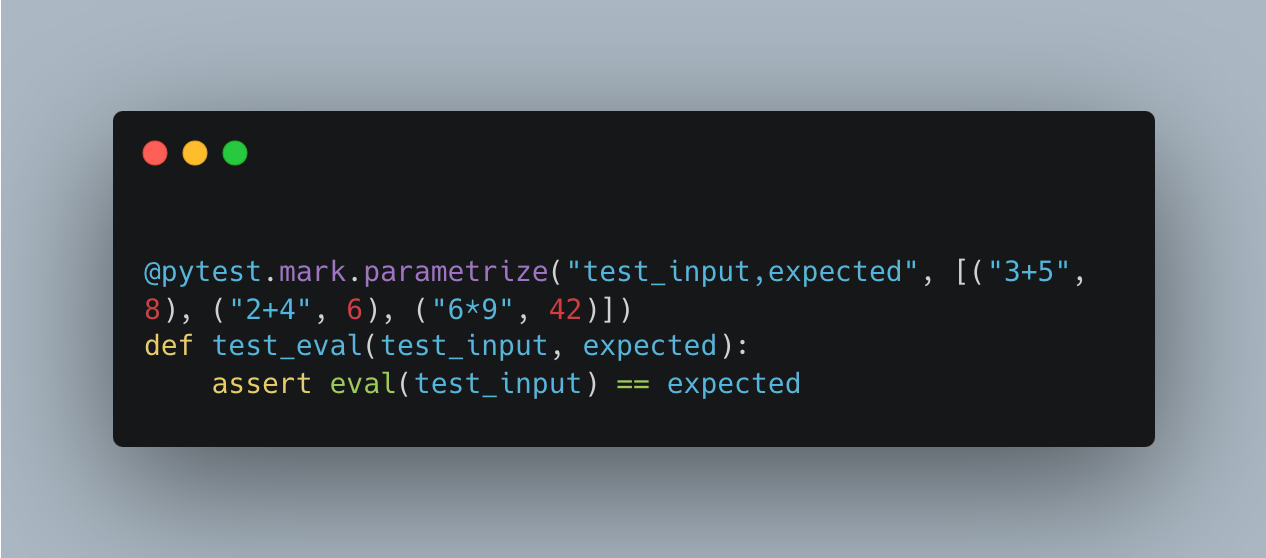
Parametrize Python
In the snippet above, the @pytest.mark.parametrize decorator defines three (test_input, expected) tuples. This allows the test_eval function to execute three times, once for each input pair, ensuring all scenarios are tested efficiently.
4. Monitor and Improve Test Coverage
Using tools like coverage.py, you can identify areas within your codebase that can benefit from tests. It ensures you have a reliable way of tracking code changes and corresponding tests.
Regularly reviewing and refactoring your codebase ensures that your tests remain effective and your code stays clean. Implementing secure code review practices can help identify potential vulnerabilities early in the development process.
5. Streamline Unit Testing with AI Agents
An AI agent is software designed to perform tasks intelligently and autonomously, mimicking human decision-making.
Key Characteristics:
- Autonomy: Operates independently without constant guidance.
- Adaptability: Continuously learns and improves based on data, feedback, and evolving testing requirements, ensuring better accuracy over time.
- Task-Specific: Tailored to specific goals like testing or analysis.
- Context-Aware: Understands and responds to its environment.
- Goal-Oriented: Efficiently achieves defined objectives.
For example, EarlyAI as an AI agent is purpose-built for generating unit tests. It adapts dynamically by analyzing your code, learning from test patterns, and refining its suggestions based on feedback. Doing so ensures increasingly precise test cases, identifies coverage gaps, and even executes validations—streamlining the development process while improving code reliability.
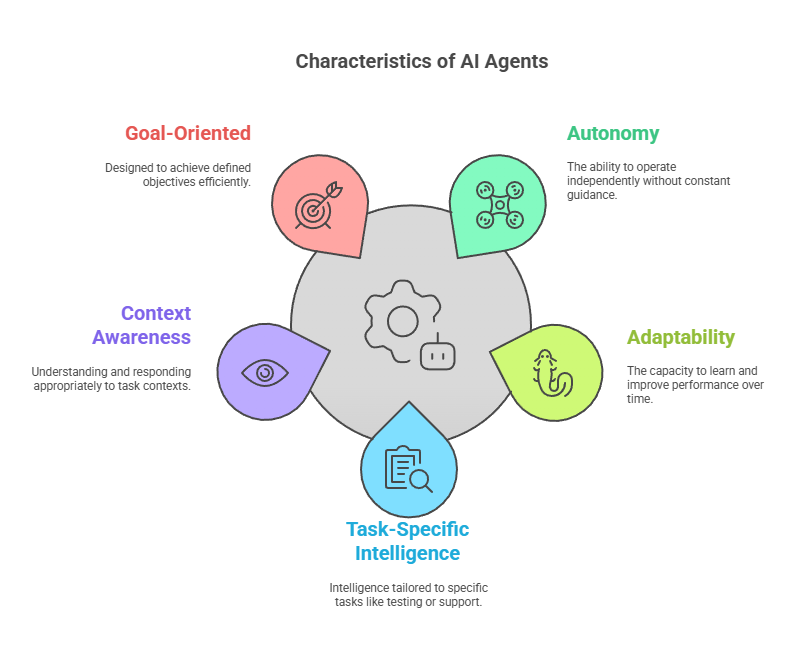
Characteristics of AI Agents
Unit testing provides peace of mind when changing your codebase, ensuring each unit functions as expected. However, the initial investment required to write comprehensive tests can be substantial.
With EarlyAI, you can confidently ship tests as quickly as you write code. AI-powered, EarlyAI understands the full context of your codebase, goes beyond the fundamental suggestions, and provides a working code project. Add it to VSCode today.