React Unit Testing: A Developer’s Guide
Ever written a React unit test that passed today and failed tomorrow for no apparent reason? You’re not alone.
46.5% of test flakiness is caused by environmental or resource-related issues like async timing and state conditions. Many developers often struggle with React unit tests since they need to mock dependencies, manage states, test asynchronous logic, and deal with tests that break even with a minor code change. These challenges slow down the development process and reduce your trust in the testing process.
Still, reliable unit tests aren’t optional if you want to move fast without breaking things. They make releases safer, refactors easier, and teams faster. Let’s break down how to make React unit testing less painful and more effective.
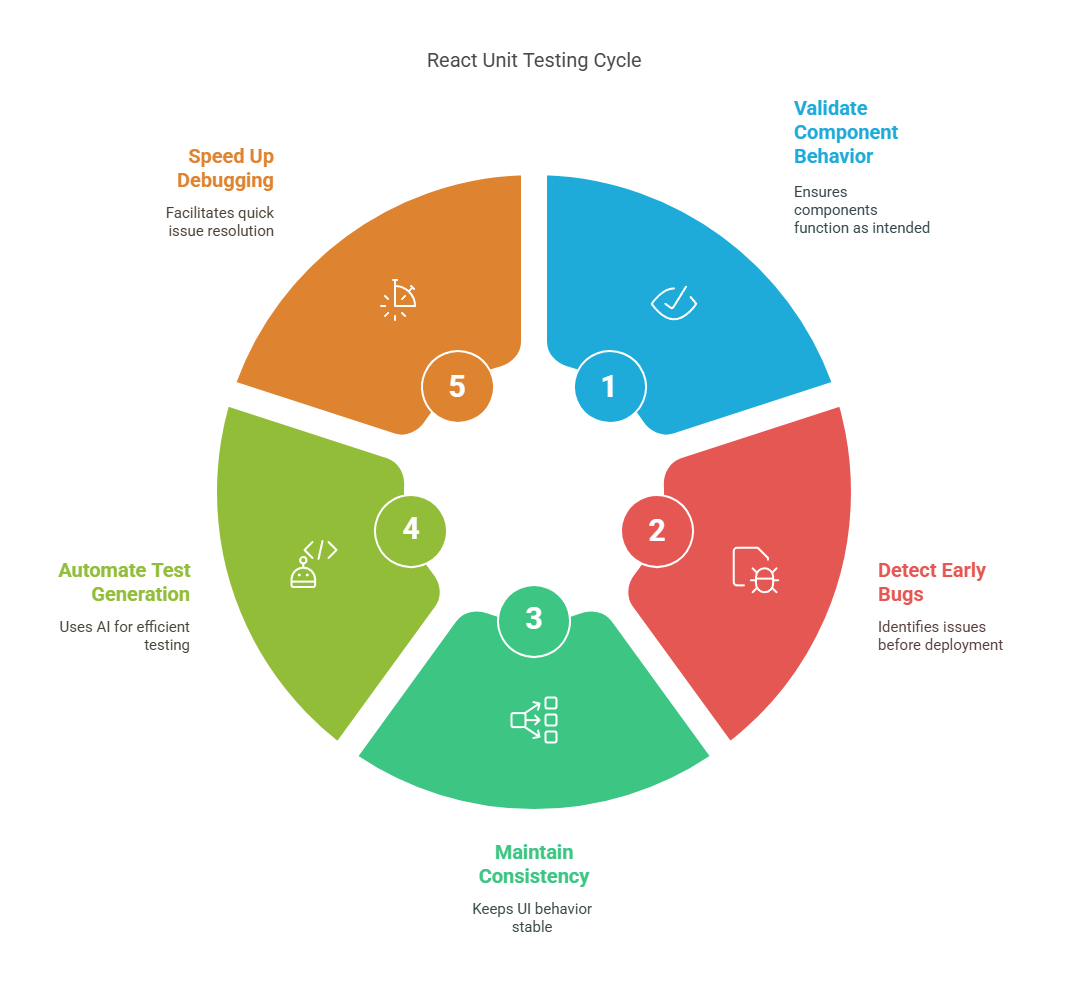
React unit testing and why it matters
What is React Unit Testing and Why Does It Matters
Unit testing in React validates that individual components behave as expected. While this sounds simple in theory, React’s dynamic nature, stateful logic, side effects, hooks, and prop-driven behavior make effective testing essential for long-term maintainability.
A single missed edge case in state logic or an incorrect prop value can quietly introduce a bug into production. Take, for example, a date picker component that fails to disable past dates after a refactor. If a test doesn’t back the logic, it might go unnoticed until it impacts real users.
Unit tests help detect these issues early, especially as your codebase grows. They offer more than just correctness; they provide safety, speed, and confidence.
Ensures UI Behavior Consistency
React does a good job hiding the DOM, but small changes—like updating a prop or adjusting a useEffect—can still mess with how a component behaves. Unit tests give you a safety net, ensuring things stay consistent when the same props or state are passed in.
Prevents Regressions During Refactors
React components often face changes like splitting components and renaming props. Although these are small changes, they can accidentally break functionalities. Unit tests allow you to verify the behavior of the application even after the slightest change.
Speeds Up Debugging and Development
Fast-failing unit tests save you from clicking around the browser just to spot bugs—automated testing strategies can push this even further by removing manual coverage bottlenecks. If you're doing TDD, they also help you write cleaner, more predictable code from the start.
Writing and maintaining tests manually becomes less practical as components evolve or rely on asynchronous logic. Agentic AI tools like EarlyAI can automate the generation of both passing and failing tests, helping teams surface edge cases early and shorten debugging cycles.
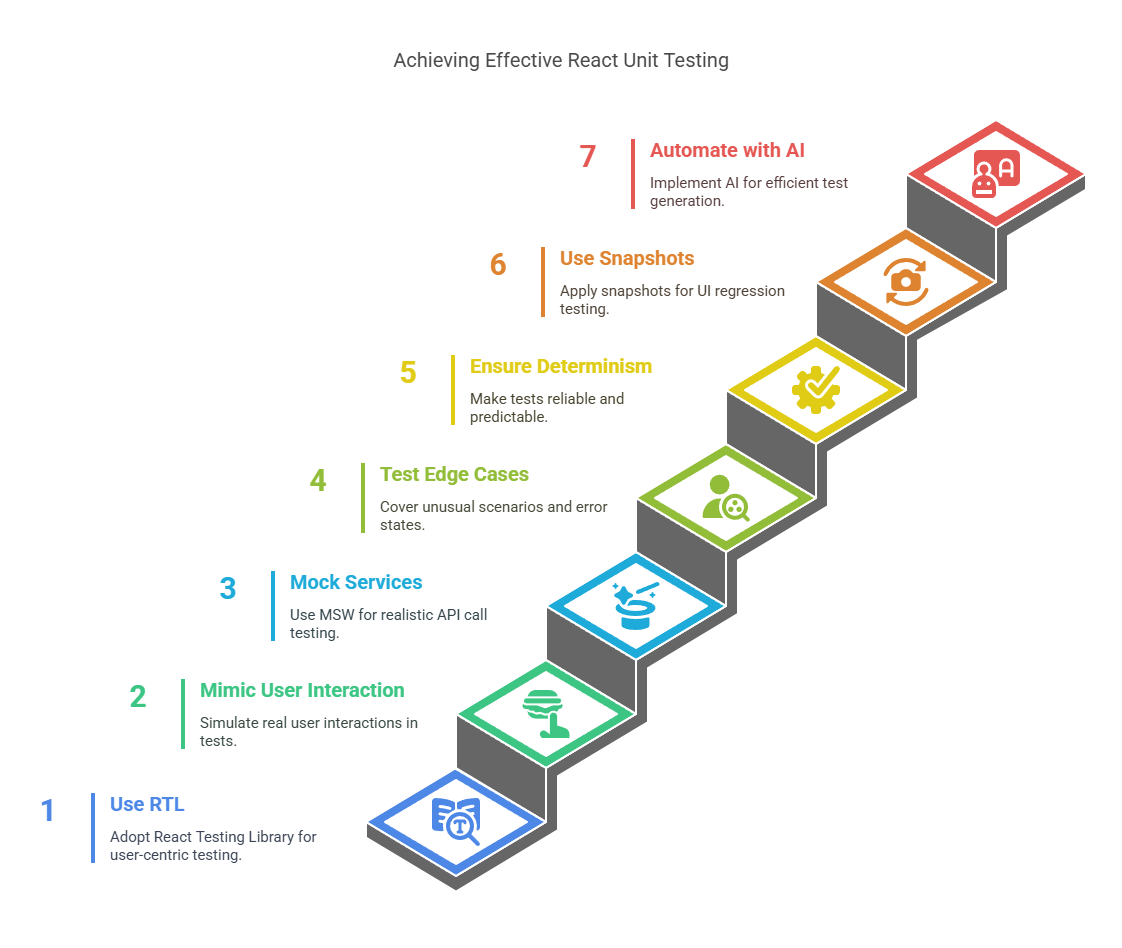
7 point checklist for effective react unit testing.
7 React Unit Testing Best Practices
These best practices are here to help you write tests that actually hold up—reliable, easy to maintain, and built to grow with your app.
1. Use React Testing Library Over Enzyme
React Testing Library (RTL) has become the standard for unit testing React components. RTL emphasizes testing components as users would interact with them. This results in more robust, future-proof tests that survive internal refactors.
import { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders the welcome message', () => {
render(<MyComponent />);
expect(screen.getByText(/welcome/i)).toBeInTheDocument();
});
2 . Write Tests That Mimic User Interaction
Avoid directly calling component methods or manipulating states to make test cases similar to real user behaviour. Testing the way a user interacts with the app makes your tests more reliable, catches side effects, and better reflects how the app is actually used.
import { render, screen, fireEvent } from '@testing-library/react';
import Counter from './Counter';
test('increments count when button is clicked', () => {
render(<Counter />);
fireEvent.click(screen.getByText('Increment'));
expect(screen.getByText('Count: 1')).toBeInTheDocument();
});
3. Mock External Services and API Calls Effectively
You can use Mock Service Worker (MSW) to mock API calls at the network level. This is really helpful when you test a component that depends on external data. Unlike mocking fetch or axios directly, MSW makes your tests more realistic and keeps them separate—a useful pattern especially as AI governance standards increasingly require auditability and traceability in test environments.
// handlers.js
import { rest } from 'msw';
export const handlers = [
rest.get('/api/user', (req, res, ctx) => {
return res(ctx.json({ name: 'Alice' }));
}),
];
// Test
test('displays fetched user name', async () => {
render(<UserProfile />);
expect(await screen.findByText('Alice')).toBeInTheDocument();
});
4. Test Edge Cases and Error States
Don’t just test when everything goes right. Make sure to cover the weird stuff too—missing props, empty data, loading screens, server errors. That’s where most bugs hide.
Most bugs happen in edge cases. Tests that validate these conditions are critical to building resilient applications.
test('shows loading spinner initially', () => {
render(<UserProfile />);
expect(screen.getByRole('status')).toHaveTextContent(/loading/i);
});
test('shows fallback when user is not found', async () => {
render(<UserProfile userId="unknown" />);
expect(await screen.findByText(/user not found/i)).toBeInTheDocument();
});
5. Make Tests Deterministic
Brittle tests are unreliable and make you lose trust in your test suite. To avoid them, don’t rely on random values, loose timeouts, or guesses about when async code finishes. Instead, use React Testing Library tools like waitFor or findBy to wait for elements properly and keep your tests stable.
test('displays success message after save', async () => {
render(<SaveForm />);
fireEvent.click(screen.getByText('Submit'));
expect(await screen.findByText(/saved successfully/i)).toBeInTheDocument();
});
6. Use Snapshot Testing Strategically
In some systems, extending test logic with runtime call durability can surface regressions that snapshots miss. Large, noisy snapshots add little value and break easily on layout changes. Use them only for pure presentational components with predictable output.
Targeted snapshots improve confidence without bloating your test suite.
import { render } from '@testing-library/react';
import renderer from 'react-test-renderer';
import Badge from './Badge';
test('renders badge correctly', () => {
const tree = renderer.create(<Badge type="success" />).toJSON();
expect(tree).toMatchSnapshot();
});
7. Automate Unit Test Generation with AI
Even with best practices, writing and maintaining unit tests is time-consuming and repetitive. Automated unit test generation with agentic AI like EarlyAI solves multiple challenges:
- Scales test creation across large or legacy codebases
- Increases coverage with green tests for current behavior and red tests for edge case failures
- Improves developer velocity by eliminating the boilerplate around test writing, aligning with key developer productivity benchmarks.
- Keeps tests up-to-date with code changes using static analysis and smart suggestions
Because EarlyAI runs inside your IDE (like VS Code), it eliminates the need for context switching. You stay in flow while tests are created automatically, making it feel like a pair programmer focused solely on testing.
Top Tools and Libraries for React Unit Testing
Here are some of the most popular tools for writing React unit tests.
Early AI
EarlyAI is a VS Code extension that automates unit test generation and maintenance for TypeScript testing tips, JavaScript, and Python..
- Generates green tests to lock in expected behavior
- Generates red tests to surface bugs and edge cases
- Keeps tests in sync as your code evolves
Why use it? It removes the overhead of writing tests by hand, boosts coverage, and helps catch regressions early—without slowing down development.
React Testing Library
RTL is built for testing React components the way users interact with them—through the UI, not internal implementation.
- Encourages tests based on real user behavior
- Reduces brittleness caused by internal refactors
- Aligns with accessibility best practices by default
Why use it? Use RTL when you want tests that reflect actual usage and hold up over time. When paired with EarlyAI, RTL becomes even more powerful—EarlyAI can auto-generate RTL-based tests, letting you skip boilerplate while still following best practices for UI-focused testing.
Jest
A fast, full-featured test runner for JavaScript.
- Supports mocking, assertions, snapshots, and coverage
- Works with React out of the box
- Integrates seamlessly with React Testing Library
Why use it? Use it to run and validate your tests efficiently in any modern React setup.
Mock Service Worker (MSW)
MSW mocks API calls at the network layer, letting components behave as if they’re hitting a real backend.
- Simulates realistic API responses
- Avoids mocking internals like fetch or axios directly
- Keeps tests clean, isolated, and closer to real-world usage
Why use it? Ideal for testing components that depend on external data without relying on live services.
Integrating React Unit Testing with CI/CD Pipelines
To actually get value from your unit tests, they need to run automatically—not just on your machine. Plugging them into a CI/CD pipeline (like GitHub Actions) makes sure every pull request is tested before it merges.
- Run tests on every pull request
- Catch regressions before they hit main
- Enforce code quality through test coverage gates
Here’s a basic GitHub Actions config to run your tests with coverage checks:
name: React CI
on: [pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: 18
- run: npm install
- run: npm run test -- --coverage
You can also configure Jest to enforce minimum coverage thresholds by adding this to package.json. Pairing this with dependency mapping techniques gives deeper insight into what parts of your app are most critical to test.
"jest": {
"coverageThreshold": {
"global": {
"branches": 80,
"functions": 80,
"lines": 80,
"statements": 80
}
}
}
With this setup, your build fails if tests break or coverage drops below 80%. That keeps your main branch clean and prevents bugs from slipping into production.
But keeping those coverage numbers up—especially as the codebase changes—can be a grind. That’s where tools like EarlyAI help out. It handles the boring stuff:
- Writes tests to boost and maintain coverage
- Flags regressions with failing test cases
- Keeps your test suite in sync as code changes
By automating the repetitive parts, EarlyAI makes it easier to stay on top of testing without slowing your team down.
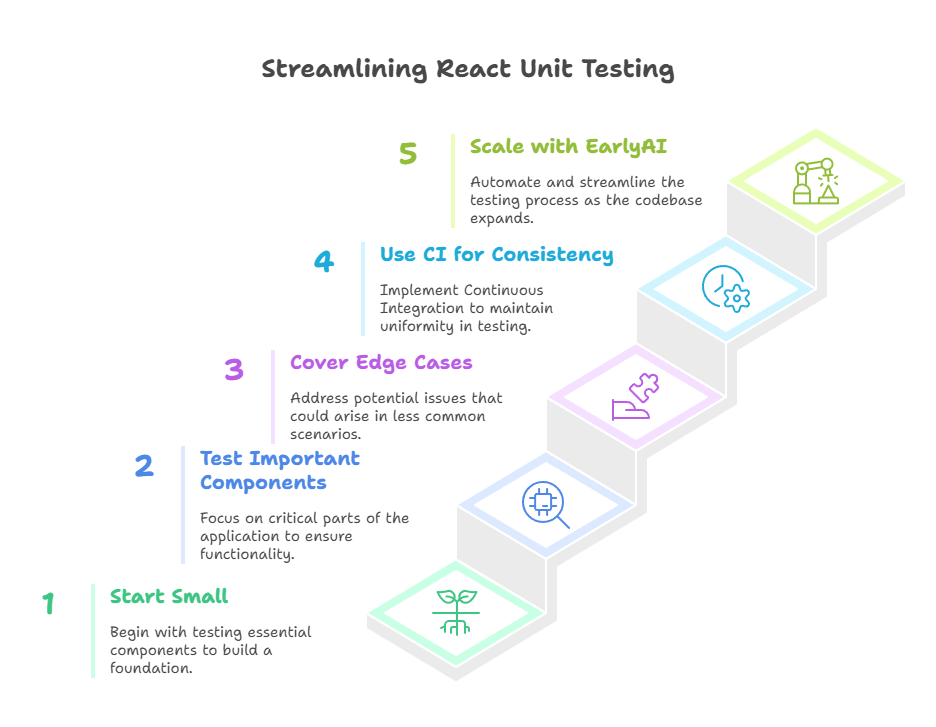
Scale your React unit tests with EarlyAI.
Ship With Confidence, Not Guesswork
React unit testing is essential if you want to move fast without breaking things. Solid tests give your team the confidence to refactor, ship quickly, and catch bugs before they reach users.
Start small. Test the important components. Cover the edge cases. Use CI to keep things consistent. And as your codebase grows, don’t try to scale all that manually.
That’s where EarlyAI helps. It handles the grunt work of writing and updating tests, so you can focus on the logic—not the boilerplate. If you're in VS Code, install EarlyAI and let it take the busywork off your plate. You’ll wonder why you ever wrote tests by hand.